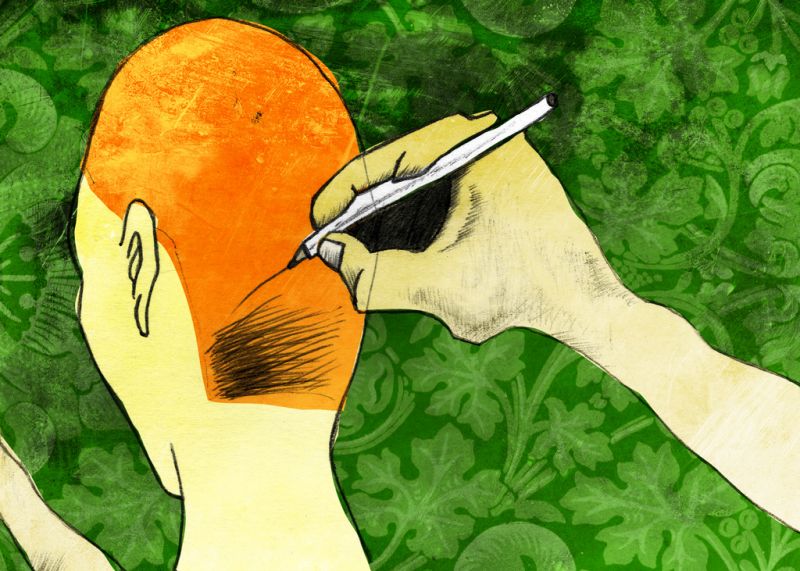
When I first got into using Sass, I investigated a lot of options.
I do a lot of work with Sitemason, so that meant that any preprocessing workflow would need to integrate with a remote service.
LiveReload ended up being a great fit. It supported Sass and Compass, but it also had an option to inject the new CSS into a remote site with the help of a web extension. I have built and launched a number of sites with LiveReload, but I wanted to experiment with some of the other Sass related technologies out there.
Namely, I wanted to try out libsass, which is a version of the Sass compiler written in C, and everybody seemed really excited about how fast it worked. To be honest, I didn’t have any complaints about the speed of LiveReload, but heck, why not give it a shot.
Prior to this adventure, I was given access to Takana. I was never able to get it to work properly with my existing Sass workflow, so I put it the shelf for a time. Now that I was starting a new project, it popped back into my mind. Takana is currently in beta, but it promises real-time Sass rendering and already uses libsass, so it seemed like a perfect time to give it another shot.
My setup also uses Grunt, which is a JavaScript task runner. Because of my remote workflow requirement, I could never use CodeKit, but I like to think of Grunt as a kind of roll-your-own CodeKit. The number of plugins available make it easy to create a really customized build process for your projects.
Setting up Grunt
- Install Node
http://nodejs.org
There are packages available for most platforms, installation was very simple on my Mac. - In the project directory, create a file called “package.json”
{ 'name': 'test', 'devDependencies': { 'grunt': 'latest', 'grunt-contrib-watch': 'latest', 'grunt-takana': 'latest' } }
This file tells Node what modules to install in the directory. Here we are loading Grunt, a watch module, and then the Takana module.
- Create Gruntfile.js
module.exports = function(grunt) { grunt.initConfig({ pkg: grunt.file.readJSON('package.json'), watch: { sass: { files: ['*.scss'], tasks: ['takana:dist'] } }, takana: { dist: { options: { outputStyle: 'compressed' }, files: { 'test.css' : 'test.scss' } } } }); grunt.loadNpmTasks('grunt-contrib-watch'); grunt.loadNpmTasks('grunt-takana'); grunt.registerTask('default', ['watch']); };
This defines a series of tasks for Grunt. In the watch command, we are telling Grunt to watch “scss” files and then run the “takana” task. That task is then going to output compressed css for the file and source that we are setting.
Something to keep in mind is that Grunt isn’t actually needed for Takana to work properly, more on that later. We do, however, need Grunt to compile our CSS at some point. Takana does offer a build option, but it wants to output CSS to a specific location. With Grunt we have a bit more control over where our files get generated, plus we can include other tasks if needed, like optimizing images or minifying JavaScript.
- In a terminal inside the project folder directory, run:
sudo npm install
This tells the node package manager to go out and download the modules we defined in “package.json,” a.k.a. magic. Also install the Grunt CLI module:
sudo npm install -g grunt-cli
- With Takana running, in the terminal type:
grunt install_takana
This connects your project directory to the Takana application. You have the option of either adding the code snippet generated to your file, or installing the web extension. I personally prefer the web extension because that way I’m not pushing live code with a localhost script tag.
- Still in terminal:
grunt
If everything is set up correctly, you should see “Waiting…” in the terminal and when you make any changes to your SCSS file and save them, the CSS should be generated right away.
Setting up Takana
Takana needs Sublime Text 3, and upon installing the program, it will add its own plugin.
In Chrome, load up your project, for me this is usually a remote site. If using the web extension, Takana will place an icon the URL bar, click that and then click the project name you added, this will associate the URL with the Takana program.
Whether using the snippet or the extension, on page load you should see a green checkmark pop up in the lower left of the screen when Takana loads correctly. If you’re really a pro, any change you now make to your SCSS is reflected live on your site, meaning as you type, you are seeing your SCSS rendered instantly in the browser, no need to even save.
If you’re like me, however, and it isn’t working yet, here are some of the gotchas I’ve encountered.
- Make sure you have a link to your CSS in your file, I think Takana needs this to know what SCSS file to live preview.
- Sometimes Takana watches the local CSS file instead of the SCSS file. Add your CSS file to the “.takana-ignore” file to avoid that.
- Libsass is picky about “@extend” so I had to change to “@include.”
- Libsass also doesn’t support Compass, but Bourbon gets me nearly everything I was using in Compass anyway, and it works great with libsass.
- Bourbon is picky about which mixins it includes, so I had to quit using them for some things like border-radius and box-shadow, which is probably a non-issue based on browser support.
- View the logs in the Takana application for helpful hints as to why things might not be working.
Generally I have found it easiest to try and get this working with a simple, blank project. Trying to add it to an existing project made me think the whole system didn’t work, when in reality it was probably my setup at the time. Building this in to a brand new site and then testing it with a really basic HTML file is a good way to start.
To set up new projects now, I just copy my Gruntfile.js and package.json to a new folder, then run “npm install,” “grunt install_takana,” “grunt,” and I’m ready to go. I don’t even need to have Grunt running most of the time, I just use it when I want to create the CSS to upload to the server, otherwise I am working entirely locally aside from the dynamic files that need to be on the server to work.
With this setup, I am getting back to the days of CSSEdit and Espresso, days when I thought I could never leave those programs because of their powerful live previewing. Sass was powerful enough to lure me away, and with these new tools, I have a feeling I’ll be here for a while.